How to Create Interactive HTML Prototypes – How Far Can You Go Without JavaScript?
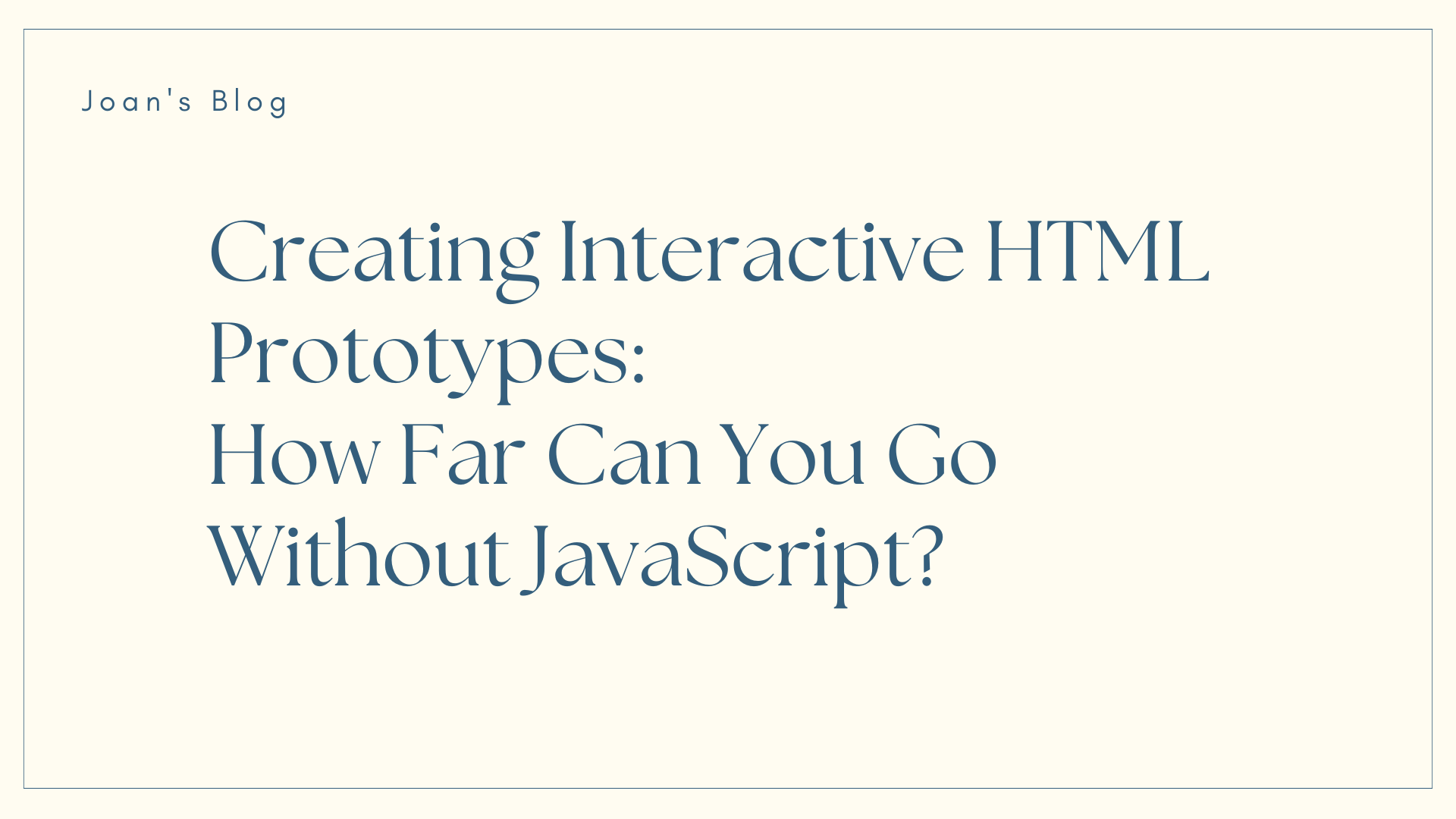
[ad_1]
Interactivity is what makes a website come alive. Whether it’s a button that reveals more content or a form that responds to your input, these little touches keep users engaged. Traditionally, we’ve relied heavily on JavaScript to make websites interactive. But what if I told you that HTML alone can do more than you think?
In this article, we’ll explore how far you can go in creating interactive prototypes using just HTML. We’ll challenge the common belief that JavaScript is always necessary for interactivity by showing you how to build engaging features with nothing but HTML. By the end, you’ll see that sometimes, simplicity is all you need to bring your ideas to life.
Table of Contents
Basics of HTML Forms
HTML forms are essential for collecting user input, whether it’s for signing up for a service, submitting feedback, or conducting a search. The power of forms lies in their ability to handle user data and send it to a server for processing. Understanding how to construct a basic form is the first step in leveraging HTML’s interactive capabilities.
A basic form in HTML might look like this
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" na<button type="submit">Submit</button>
</form>
-
The
<form>
element is a container for all the input elements. Theaction
attribute specifies where the form data should be sent when the form is submitted. Themethod
attribute determines how the data is sent (typically usingGET
orPOST
). -
The
<label>
element provides a user-friendly label for each input field, improving accessibility. -
The
<input>
elements are where users enter their information. Each input has atype
attribute that defines what kind of data it accepts (for example:text
,email
,password
). -
The
<button>
element submits the form when clicked.
Forms are fundamental because they involve direct user interaction, making them a critical component of any interactive prototype.
Interactive HTML Elements
Beyond forms, HTML includes a variety of elements that naturally respond to user actions. These elements can be used to create interactive interfaces without writing any JavaScript, which is perfect for quick prototyping.
Buttons, Checkboxes, and Radio Buttons
Buttons: Buttons are one of the most straightforward interactive elements. They can perform a wide range of actions, from submitting a form to triggering a CSS animation. Buttons are usually defined with the <button>
or <input type="button">
elements.
<button type="button">Click Me</button>
In the above example, the button doesn’t do anything by itself unless tied to a form or an action. However, in prototypes, buttons can visually represent functionality, making the prototype feel more complete.
Checkboxes: Checkboxes allow users to select multiple options from a list. They are ideal for scenarios where more than one choice is allowed.
<input type="checkbox" id="option1" name="option1" value="Option 1">
<label for="option1">Option 1</label>
Each checkbox can be checked or unchecked, providing immediate visual feedback.
Radio Buttons: Radio buttons are used when only one option can be selected from a group. This is useful in cases like surveys or quizzes where the user needs to choose one answer.
<input type="radio" id="choice1" name="choice" value="Choice 1">
<label for="choice1">Choice 1</label>
<input type="radio" id="choice2" name="choice" value="Choice 2">
<label for="choice2">Choice 2</label>
When one radio button in a group is selected, the others are automatically deselected, ensuring that only one option is chosen.
These elements are simple yet powerful, providing the basic building blocks for interactive user interfaces.
The <details>
and <summary>
Elements
The <details>
and <summary>
elements provide a way to create sections of content that users can expand or collapse. This is particularly useful for FAQs, where you might want to show the question and hide the answer until the user chooses to reveal it.
<details>
<summary>What is HTML?</summary>
<p>HTML stands for HyperText Markup Language. It is the standard language used to create web pages.</p>
</details>
What is HTML?
HTML stands for HyperText Markup Language. It is the standard language used to create web pages.
How it works:
-
The
<summary>
element is the clickable heading that the user interacts with. -
The content inside the
<details>
tag (but outside the<summary>
tag) remains hidden until the user clicks on the summary, revealing it.
This simple interaction adds a layer of user control, allowing content to be hidden or shown based on user action, all without JavaScript.
Different Types of <input>
The <input>
element is one of the most versatile in HTML. Depending on its type
attribute, it can serve different purposes, from accepting text input to allowing date selection. Understanding the various input types is key to creating functional prototypes.
Here are a few common types:
Text Input: This is the most common input type, used for single-line text input.
<input type="text" name="username" placeholder="Enter your username">
The placeholder
attribute provides a hint to the user about what to enter.
Password Input: This input type hides the characters as the user types, making it suitable for sensitive information like passwords.
<input type="password" name="password" placeholder="Enter your password">
Email Input: The email
type ensures that the user’s input is in a valid email format.
<input type="email" name="email" placeholder="Enter your email">
Date Input: This type provides a date picker, allowing users to select a date from a calendar interface.
<input type="date" name="birthdate">
These different input types enhance user experience by providing specialized interfaces for different types of data. They help make the form more intuitive and reduce the likelihood of user errors.
How to Create Advanced Interactive Prototypes with HTML
Once you’re comfortable with the basics of HTML forms and interactive elements, you can start creating more advanced prototypes that offer a richer user experience. In this section, we’ll explore how to combine form elements to build complex interactions and simulate dynamic content updates, using only HTML.
How to Combine Form Elements for Complex Interactions
Combining different form elements can help you create more sophisticated interactions and user experiences. While HTML alone has its limitations, you can still achieve quite a bit by using these elements creatively.
Multi-step Forms and Conditional Inputs
Multi-step forms are useful when you want to break a long form into smaller, more manageable sections. This approach can improve user experience by making complex forms feel less overwhelming. While HTML alone doesn’t support multi-step functionality directly, you can use the <fieldset>
and <legend>
elements to organize form sections visually.
Example of a Multi-step Form:
<form>
<fieldset>
<legend>Step 1: Personal Information</legend>
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<button type="button" onclick="document.getElementById('step2').style.display='block';">Next</button>
</fieldset>
<fieldset id="step2" style="display:none;">
<legend>Step 2: Address Details</legend>
<label for="address">Address:</label>
<input type="text" id="address" name="address">
<label for="city">City:</label>
<input type="text" id="city" name="city">
<button type="button" onclick="document.getElementById('step2').style.display='none';">Previous</button>
<button type="submit">Submit</button>
</fieldset>
</form>
How it works:
-
Fieldsets: The
<fieldset>
element groups related fields together and helps visually separate different sections of the form. -
Legends: The
<legend>
element provides a title for each section. -
Buttons: Use
<button>
elements withonclick
attributes to show or hide sections. In a real-world scenario, you’d use JavaScript for better control, but this example demonstrates the concept.
Conditional Inputs allow users to fill out additional fields based on previous choices. While HTML alone doesn’t support this functionality natively, you can use checkboxes and radio buttons creatively to show or hide sections of the form.
Example of Conditional Inputs:
<form>
<label for="subscribe">
<input type="checkbox" id="subscribe" name="subscribe">
Subscribe to newsletter
</label>
<div id="newsletterDetails" style="display:none;">
<label for="frequency">Preferred Frequency:</label>
<select id="frequency" name="frequency">
<option value="weekly">Weekly</option>
<option value="monthly">Monthly</option>
</select>
</div>
<script>
document.getElementById('subscribe').addEventListener('change', function() {
document.getElementById('newsletterDetails').style.display = this.checked ? 'block' : 'none';
});
</script>
</form>
How it works:
-
Checkboxes: The checkbox lets users opt into additional options.
-
Conditional Display: The
<div>
withid="newsletterDetails"
shows or hides based on the checkbox state. While the JavaScript is necessary to handle the condition, HTML alone provides the structure and initial display.
Simulating Dynamic Content Updates
Simulating dynamic content updates involves creating sections of a webpage that can change based on user input. While full dynamic updates require JavaScript, you can use HTML and CSS to simulate these changes.
Example of Simulated Dynamic Content Updates:
<form>
<label for="view">Choose a view:</label>
<select id="view" name="view">
<option value="overview">Overview</option>
<option value="details">Details</option>
</select>
<div id="overviewContent">
<h2>Overview</h2>
<p>This is the overview content.</p>
</div>
<div id="detailsContent" style="display:none;">
<h2>Details</h2>
<p>This is the detailed content.</p>
</div>
<script>
document.getElementById('view').addEventListener('change', function() {
var selectedView = this.value;
document.getElementById('overviewContent').style.display = selectedView === 'overview' ? 'block' : 'none';
document.getElementById('detailsContent').style.display = selectedView === 'details' ? 'block' : 'none';
});
</script>
</form>
How it works:
-
Dropdown Menu: A
<select>
element allows users to choose between different views. -
Content Sections: The content sections are shown or hidden based on the user’s selection. This simulation relies on JavaScript to handle the visibility, but the structure is set up with HTML.
While true dynamic updates are best handled with JavaScript, these examples show how you can create the illusion of interactivity using HTML and CSS alone.
HTML-Only Interactive Techniques
While JavaScript is commonly used for creating interactive features, HTML itself offers several techniques to achieve interactivity. In this section, we’ll explore four HTML-only techniques: using the target
attribute for page-level interactions, emulating modal dialogs, creating tooltips, and building interactive image maps. Each technique highlights how HTML can be used creatively to add interactive elements to your webpages.
How to Use the target
Attribute for Page-level Interactions
The target
attribute allows you to control where a linked document will open. This can be used to create interactive experiences that involve navigating between different sections of a page or opening new pages within the same window or tab.
Example of Using the target
Attribute:
<a href="#section1" target="_self">Go to Section 1</a>
<a href="#section2" target="_self">Go to Section 2</a>
<h2 id="section1">Section 1</h2>
<p>Content for Section 1...</p>
<h2 id="section2">Section 2</h2>
<p>Content for Section 2...</p>
How it works:
-
Anchors (
<a>
elements): These links navigate to different parts of the same page or other pages. Thehref
attribute points to the target location. -
target="_self"
: This attribute ensures the link opens in the same window or tab, which is the default behavior. You can use other values like_blank
to open links in a new tab or window.
Using the target attribute allows you to control how users interact with links and navigate your site, enhancing the user experience without relying on JavaScript.
How to Emulate Modal Dialogs
Modal dialogs are often used to display important information or prompts that require user interaction before proceeding. While creating true modals typically involves JavaScript, you can use HTML and CSS to simulate modal dialogs.
Example of Emulating a Modal Dialog:
<input type="checkbox" id="modal-toggle">
<label for="modal-toggle" style="cursor: pointer;">Open Modal</label>
<div class="modal">
<div class="modal-content">
<a href="#" class="close" onclick="document.getElementById('modal-toggle').click();">×</a>
<h2>Modal Title</h2>
<p>This is a simple modal dialog without JavaScript!</p>
</div>
</div>
<style>
#modal-toggle {
display: none;
}
.modal {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
justify-content: center;
align-items: center;
}
#modal-toggle:checked ~ .modal {
display: flex;
}
.modal-content {
background-color: white;
padding: 20px;
border-radius: 5px;
max-width: 500px;
width: 100%;
text-align: center;
position: relative;
}
.close {
position: absolute;
top: 10px;
right: 10px;
font-size: 24px;
text-decoration: none;
color: #333;
cursor: pointer;
}
.close:hover {
color: #ff4c4c;
}
</style>
How it works:
-
Checkbox (
<input type="checkbox">
): Used to control the visibility of the modal. -
Labels: The labels act as buttons to open and close the modal. Clicking the “Open Modal” label checks the checkbox, which makes the modal visible. Clicking the “Close” label unchecks the checkbox and hides the modal.
-
CSS: Controls the appearance and positioning of the modal and its overlay.
This approach allows you to create a modal-like effect with HTML and CSS alone, providing a user-friendly way to present information or options.
How to Create HTML-only Tooltips
Tooltips provide additional information when a user hovers over an element. While JavaScript can enhance tooltips, you can create basic tooltips using just HTML and CSS.
Example of Creating Tooltips:
<div class="tooltip">
Hover over me
<span class="tooltip-text">Tooltip text</span>
</div>
<style>
.tooltip {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip .tooltip-text {
visibility: hidden;
width: 120px;
background-color: black;
color: #fff;
text-align: center;
border-radius: 5px;
padding: 5px 0;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
margin-left: -60px;
opacity: 0;
transition: opacity 0.3s;
}
.tooltip:hover .tooltip-text {
visibility: visible;
opacity: 1;
}
</style>
How it works:
-
Tooltip Container: The
<div class="tooltip">
contains the text that triggers the tooltip and the tooltip text itself. -
Tooltip Text: The
<span class="tooltip-text">
is hidden by default and only becomes visible when the user hovers over the tooltip container. -
CSS: Uses
visibility
andopacity
to show and hide the tooltip, with a transition effect for smooth appearance.
This method allows you to add helpful hints or extra information to your webpage without needing JavaScript.
How to Build Interactive Image Maps
Image maps enable you to create clickable areas on an image, allowing users to interact with different parts of an image. HTML’s <map>
and <area>
elements are used to define these clickable regions.
Example of Building an Image Map:
<img src="map-image.jpg" usemap="#image-map" alt="Map Image">
<map name="image-map">
<area shape="rect" coords="34,44,270,350" href="page1.html" alt="Region 1">
<area shape="circle" coords="130,136,60" href="page2.html" alt="Region 2">
<area shape="poly" coords="300,50,400,150,350,200" href="page3.html" alt="Region 3">
</map>
How it works:
-
Image: The
<img>
tag includes theusemap
attribute, which links to the<map>
element. -
Map and Areas: The
<map>
element contains one or more<area>
elements. Each<area>
defines a clickable region on the image.
Image maps allow you to create interactive images with different regions that lead to various pages or actions, adding an extra layer of engagement to your prototypes.
Design Tips for HTML-Only Prototypes
Designing HTML-only prototypes involves focusing on both usability and performance. While HTML alone provides a solid foundation for interactive elements, it’s essential to ensure that your prototypes are accessible, fast, and efficient. This section offers tips on making your HTML-only prototypes more effective, covering accessibility, performance, and limitations.
Make Sure Your Prototype is Accessible
Accessibility ensures that your prototypes can be used by everyone, including those with disabilities. By adhering to accessibility principles, you improve the user experience for all users and make your prototypes more inclusive.
Keyboard-friendly Navigation
Many users rely on keyboards for navigation, so it’s crucial to ensure that all interactive elements can be accessed and used via keyboard alone.
Tips for Keyboard-Friendly Navigation:
-
Use Semantic HTML: Proper use of HTML elements like
<button>
,<a>
, and<input>
helps with keyboard navigation. These elements are naturally focusable and keyboard-navigable. -
Tab Index: The
tabindex
attribute can be used to manage the order of focusable elements. For example,tabindex="0"
allows an element to be focusable, while negative values liketabindex="-1"
remove an element from the tab order. -
Visible Focus Indicators: Ensure that focus indicators (like outlines) are visible when navigating with the keyboard. This helps users see which element is currently in focus.
Example:
<button tabindex="0">Click Me</button>
<a href="#section1" tabindex="0">Go to Section 1</a>
<input type="text" tabindex="0" placeholder="Enter text here">
Screen Reader Support
Screen readers help visually impaired users navigate your site. To ensure that your prototypes are accessible to these users, include relevant ARIA (Accessible Rich Internet Applications) attributes and use semantic HTML.
Tips for Screen Reader Support:
-
Alt Text: Use the
alt
attribute for images to provide descriptive text for screen readers. -
ARIA Roles and Labels: Use ARIA roles and labels to enhance the accessibility of interactive elements. For example,
role="button"
can be used for clickable elements that are not inherently buttons. -
Descriptive Labels: Ensure that form inputs and interactive elements have clear and descriptive labels.
Example:
<img src="logo.jpg" alt="Company Logo">
<button aria-label="Close" onclick="closeModal()">X</button>
Incorporating these practices makes your prototypes more navigable and usable for individuals with disabilities.
The Speed and Simplicity of HTML-only Prototypes
HTML-only prototypes are often faster to load and simpler to maintain due to their reliance on minimal resources. These benefits contribute to a more efficient user experience.
Faster Loading
HTML-only prototypes typically load faster because they don’t rely on external scripts or complex interactions that can slow down performance. The simplicity of HTML means fewer resources need to be processed by the browser.
Benefits:
-
Reduced Load Times: Without JavaScript or complex CSS, the browser can render the content more quickly.
-
Improved Performance: Fewer resources mean less strain on the client’s device, resulting in smoother performance.
Lower Resource Use
Using HTML alone minimizes resource consumption. Without JavaScript or heavy CSS, your prototype consumes less memory and processing power.
Benefits:
-
Lower Memory Usage: Less reliance on external scripts means reduced memory footprint.
-
Less CPU Usage: Simplified interactions reduce the need for extensive computation, leading to lower CPU usage.
These aspects contribute to a more efficient and responsive user experience, especially important for users on slower connections or less powerful devices.
Where HTML Falls Short
HTML is great for building interactive prototypes, but it has some limits. Knowing these limits helps you decide when to add other technologies.
No Complex Logic
HTML alone is limited in handling complex logic and dynamic interactions. For example, conditions, loops, or advanced calculations require JavaScript.
Limitations:
-
Conditional Logic: HTML cannot perform actions based on complex conditions or states.
-
Dynamic Updates: Changes to the page content or structure based on user input often require JavaScript.
Example Limitation:
- Interactive Forms: Complex forms with dynamic fields or conditional sections generally need JavaScript to manage the logic and interactions effectively.
Difficulties with More Advanced Interactions
Some interactions, such as real-time updates or complex animations, are challenging to achieve with HTML alone. While CSS can handle some animations, more advanced interactions often require scripting.
Limitations:
-
Real-time Data: Updating content in real time based on user actions or external data sources is typically not possible with HTML alone.
-
Complex Animations: Advanced animations and transitions usually require JavaScript or CSS animations.
Example Limitation:
- Live Content Updates: HTML cannot fetch or update content dynamically without JavaScript or server-side solutions.
Recognizing these limitations helps you balance the use of HTML with other technologies when needed to achieve your desired functionality.
When to Use HTML-Only Prototypes
HTML-only prototypes can be a powerful tool in your design toolkit, especially when simplicity and performance are key.
Understanding when to use HTML-only solutions, considering your audience, and knowing how to blend HTML with JavaScript can help you create effective prototypes that meet your project needs.
When HTML-only is the Right Choice
HTML-only prototypes are particularly useful in specific scenarios where their simplicity and efficiency shine. Here are some situations where using HTML-only prototypes makes sense:
1. Simple Forms and Surveys: When you need to create basic forms or surveys to collect user input, HTML forms with standard input elements can be used effectively. These prototypes are easy to set up and provide a straightforward way for users to submit information.
Example:
2. Static Information Display: For displaying static content or information, HTML is sufficient. Prototypes that focus on showcasing content without requiring dynamic interactions are ideal candidates for HTML-only design.
Example:
3. Prototyping Early Concepts: When prototyping early concepts or initial designs, HTML-only prototypes can help quickly visualize and test ideas without the need for complex scripting. This approach allows you to iterate rapidly and focus on layout and structure.
Example:
4. Performance Considerations: For situations where performance is critical and you need to ensure fast loading times and low resource usage, HTML-only prototypes can be advantageous. They eliminate the overhead of additional scripts and reduce processing demands.
Example:
5. Accessibility and Simplicity: When designing for accessibility and simplicity, HTML provides a strong foundation. Using semantic HTML and built-in attributes ensures that your prototypes are accessible to users with disabilities.
Example:
-
An accessible form with clear labels and input fields.
-
A simple navigation menu with clear, focusable links.
Understanding Your Audience
Knowing your audience is crucial when deciding whether to use HTML-only prototypes. Consider the following factors:
1. User Needs and Expectations: Understand what your users need and expect from the prototype. If your audience is looking for simple, straightforward interactions, HTML-only may be sufficient. For more complex interactions or dynamic content, consider integrating JavaScript.
Example:
-
Users who need a basic contact form may be satisfied with an HTML-only solution.
-
Users who expect interactive features or real-time updates might require a combination of HTML and JavaScript.
2. Technical Constraints: Consider the technical constraints of your audience’s devices and browsers. HTML-only prototypes generally perform well across various devices and browsers, making them suitable for diverse audiences.
Example:
- A prototype for users with older devices or limited internet connectivity may benefit from the simplicity of HTML-only design.
3. User Expertise: Assess the technical expertise of your audience. If they are not familiar with complex interactions or scripting, HTML-only prototypes can provide a more accessible and user-friendly experience.
Example:
- A prototype for a non-technical audience may prioritize simplicity and ease of use with HTML-only design.
4. Feedback and Iteration: Gather feedback from users to understand how they interact with your prototype. If users find HTML-only prototypes sufficient for their needs, you can continue with this approach. If more advanced features are requested, consider integrating additional technologies.
Example:
- Collect user feedback on a basic form and decide if additional features or interactions are needed.
Mixing HTML and JavaScript
While HTML-only prototypes have their strengths, combining HTML with JavaScript can enhance functionality and provide a richer user experience.
Here’s when and how to mix HTML and JavaScript effectively:
1. Adding Dynamic Interactions: When your prototype requires dynamic interactions, such as real-time updates or complex logic, JavaScript can complement HTML to provide these features.
Example:
2. Enhancing User Experience: JavaScript can be used to improve the user experience by adding interactive elements like modals, carousels, or animations that HTML alone cannot achieve.
Example:
3. Handling Complex Logic: For prototypes involving complex calculations, conditional logic, or data manipulation, JavaScript can handle these requirements more effectively than HTML alone.
Example:
4. Iterating and Testing: Start with an HTML-only prototype to establish the basic structure and layout. Once you have a clear understanding of the design, integrate JavaScript to add interactivity and test the enhanced functionality.
Example:
- Begin with a static prototype of a feature and then add JavaScript to refine and test interactive elements.
5. Balancing Complexity: Use JavaScript to enhance the prototype where necessary, but avoid overcomplicating the design. Maintain a balance between simplicity and functionality to ensure the prototype remains easy to use and understand.
Example:
- Implement JavaScript for essential interactions but keep the overall design and layout straightforward.
Conclusion
HTML-only prototypes are a great choice for many design tasks because they are simple and quick to build. They work well for basic interactions like forms and displaying information.
HTML alone is powerful for straightforward designs. However, if you need more advanced features or dynamic interactions, adding JavaScript can help. Mixing HTML with JavaScript lets you enhance your prototypes when needed.
In summary, HTML-only prototypes offer speed, accessibility, and ease of use. Understanding when to use HTML by itself and when to add other tools ensures that your prototypes are both effective and user-friendly.
That’s all for this article! If you’d like to continue the conversation or have questions, suggestions, or feedback, feel free to reach out to connect with me on LinkedIn. If you enjoyed this content, consider buying me a coffee to support the creation of more developer-friendly contents.
[ad_2]
Source link